Introduction
Borderlands is one of my favorite game franchises. I've put countless hours into each game. It's a first-person looter shooter video that takes place in Western science fiction. The gear in the game comes in different rarity levels. The better the gear, the harder it is to find. One way of acquiring gear is by using keys to open a loot chest where you have a higher likelihood of landing a rare piece of gear. To obtain these keys you have to enter SHiFT codes that Gearbox, the creator of Borderlands, releases from time to time. The only problem is that these SHiFT codes often come with expiration dates, so unless you're on the lookout, you might miss out on the code.
Making sure I never miss out
In order to make sure that I never miss a SHiFT code I started thinking of ways to alert myself when a code goes live. These codes are often released on Twitter so I could enable notifications for the accounts that tweet the codes out. But these accounts don't only tweet out codes, and I didn't want to be notified every time they tweeted about sitting on the patio.
So I thought about writing a bot that would monitor these twitter profiles for any tweet that looked like a SHiFT code and then I could have it email them or text them to myself. Thankfully, Orcicorn beat me to it. They already built a bot that monitors the likely places SHiFT codes are released and they add them to a list of codes on their website.
Sending the codes to myself automatically
Okay so now I have a place where I can easily access all the latest codes. Now I need a way of sending them to myself automatically so I can redeem them before they expire. For this part I ended up creating a simple web scraper that monitors the website that has all the codes and then once a day it checks for new codes. Then if there have been new codes added I send myself a text message with the code via Twilio. And I actually send the code in the form of a link so I can easily redeem it on the SHiFT website. I could have automated it even further and just redeemed the codes automatically without even worrying about texting them to myself, but when I checked the SHiFT code website's Code of Conduct they seemed to frown on that, and it felt a little too easy anyway. On top of that I wanted to know when I was redeeming the codes and what the codes actually redeemed. It's not always keys, sometimes the codes will redeem unique gear or skins.
Here is the code that I wrote that checks for new SHiFT codes and sends me a text message anytime new codes are found. I host this code on Napkin.io and I scheduled it to run everyday at 10:00PM UTC.
javascript
Automating code redemption
While I didn't fully automate the process of redeeming the codes, I did semi-automate it. I have the code texted to my Google phone number so I can access it from my browser on my Mac. And I have it sent to myself as a link.
uri
I've installed the Tampermonkey extension to my browser, which lets you write JavaScript that runs on whatever website you choose. So you can add functionality to a website that makes it a little easier to use. If you look at the link above you'll notice the query string code=t3fjt-sbzzt-93bfc-3b3tb-3btrb
. Using Tampermonkey I wrote JavaScript that runs every time I load the SHiFT website on my browser and it checks for the value assigned to code
.
javascript
The JavaScript function above will find the code in the url and fill out the form on the SHiFT website automatically.
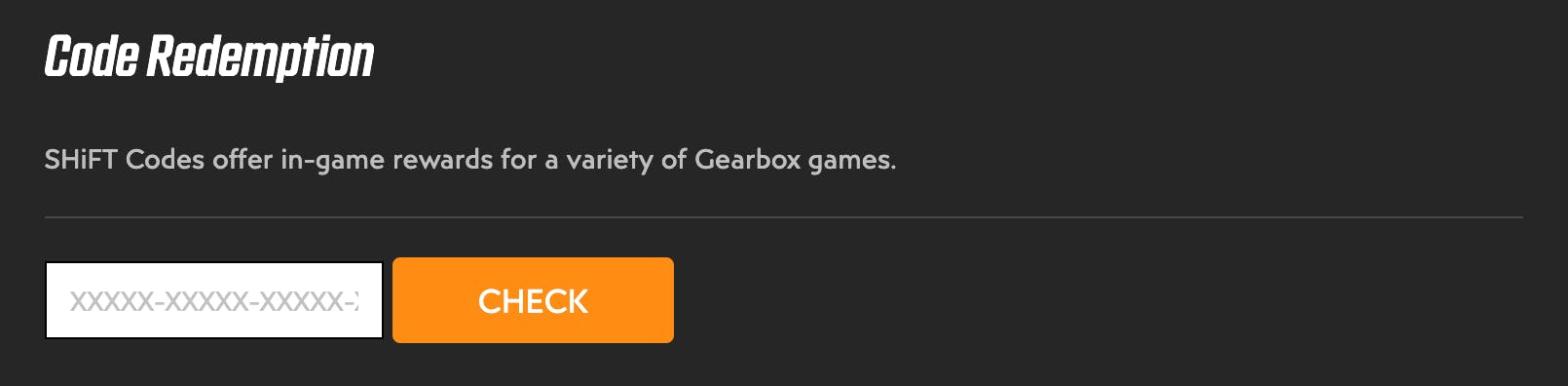
This is slightly different from the automatic redemption because it only works when I manually load the website with a specific url, and it only works when I've manually logged in to the website.
Results
This system that I've built has been extremely beneficial to my gaming endeavors. Thanks to this setup I've been able to easily redeem over 100 SHiFT codes. And the keys that I've obtained from these codes have helped me buy gear that has helped me saves lives and earn millions of dollars (in the game not in real life unfortunately).