Introduction
I'm currently building a WordPress website for a company that rents out equipment. They want to allow their customers to rent equipment from their website, rather than having to call or stop in at the shop.
A couple years ago I built a website for a company that has studios that photographers can book for photo sessions. To add the booking functionality to this website I used the WooCommerce Bookings And Appointments plugin by PluginHive. I was planning on using this plugin again for the equipment rental company but the issue I was facing is that they want their website to work directly with their ERP, and this is pretty difficult to accomplish using a third-party plugin.
So, in order to add the booking functionality that integrates directly with the ERP I built a custom datepicker plugin using Svelte.
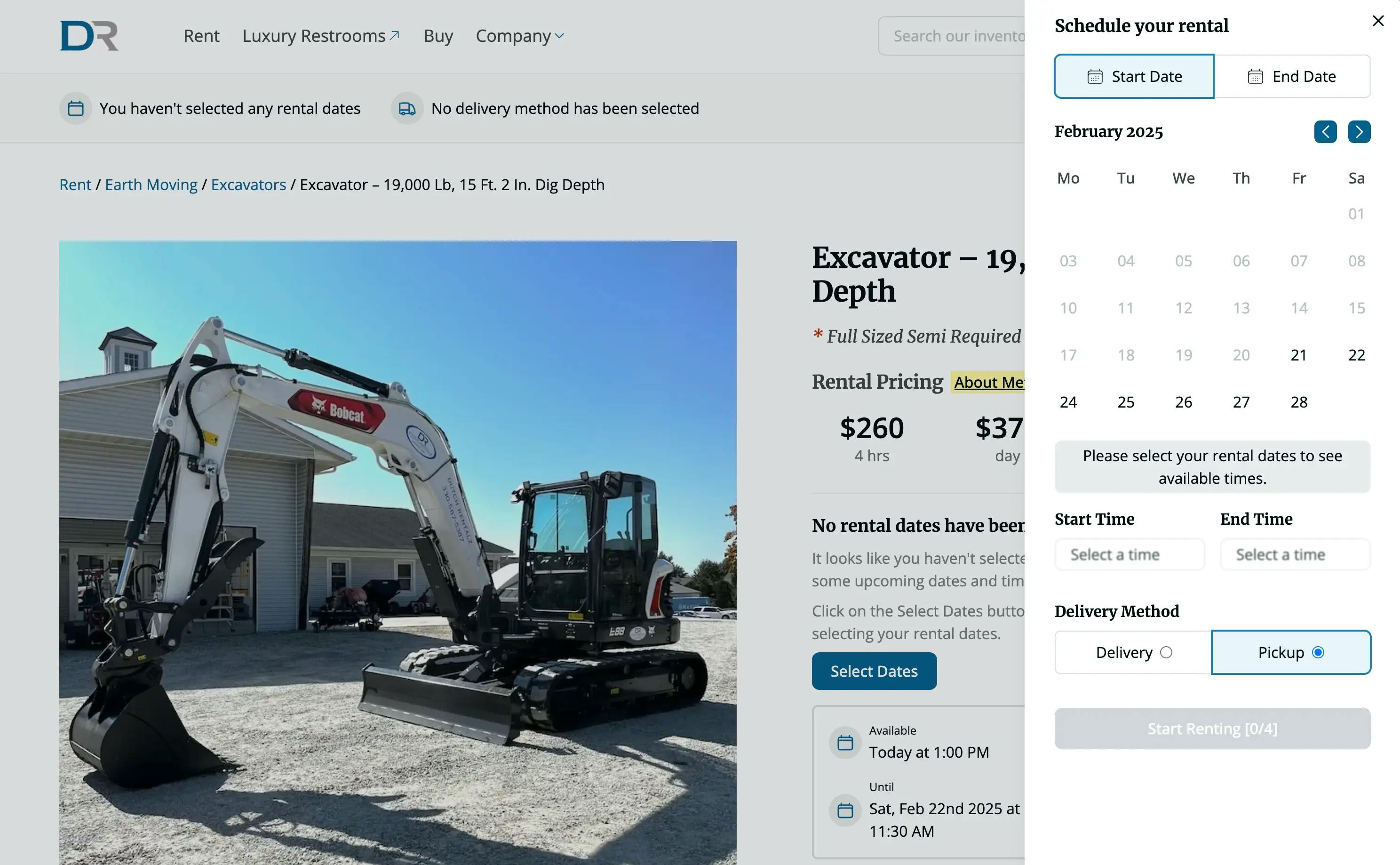
Building the datepicker
This post walks through a basic datepicker built with Svelte. The plugin I built for the equipment rental company is quite a bit more complex but this is a good starting point.
Define the basic calendar grid
We start by defining a general grid for a calendar month and initializing the grid with let rows = initRows()
. This initRows function just describes how many days are in a week, weeks in a month, etc. We'll fill this out with data for the selected month and year in a minute.
javascript
Fill out the calendar grid for the selected month and year
Next we fill out the calendar grid with the correct dates using the components onMount
lifecycle function.
javascript
Updating the calendar grid
Now whenever we change the month and/or year we also need to update the calendar grid.
javascript
Displaying the calendar
Now that we have an array of days for the selected month and year we can display them in the calendar.
svelte
View the project
For a full look at how this basic datepicker was created you can check out the GitHub repo. To see the datepicker in action check out the link below! Keep in mind this is only meant to be a starting point that you can build off so it is pretty basic, but it can be customized pretty extensively to meet your needs.
GitHub Repository
https://github.com/zpthree/svelte-datepicker