Introduction
In this walkthrough, we'll be using Shopify CLI to initialize a new Shopify theme and run the Shopify development server. We'll install Tailwind CSS inside of our Shopify theme, and we'll install concurrently to allow us to run multiple commands at the same time.
Setting up a new theme
Everything in this section can be found in Shopify's theme documentation. If anything is unclear in this walkthrough or something isn't working be sure to check out their docs.
If you don't already have a theme set up you can initialize a new theme by running the following command in your terminal:
bash
You will be prompted to give your theme a name so make sure you name it something cool, like siberian-snowman
.
Once your theme is downloaded you can navigate to the theme folder in your terminal by running the following. Just make sure you replace my cool theme name with your own.
bash
Running the dev server
To start the Shopify dev server you can run shopify theme dev
.
"The command returns a URL that hot reloads local changes to CSS and sections, allowing you to preview changes in real time using the store's data. This preview is only available in Google Chrome."
Starting the Shopify dev server for the first time
The first time you start the dev server you'll want to run the following command replacing {store-name}
with the name of your store. This will make sure that the store you specify gets used to preview your new theme.
bash
Once you run this command you will be asked to log in to your Shopify store.
After you start the dev server for the first time you can just run shopify theme dev
to start the dev server. The store that you just specified will now get used automatically when the dev server starts (unless you change the active store for any reason).
Adding Tailwind CSS to your new theme
Now that we have a Shopify theme we can finally get Tailwind CSS added. Adding Tailwind CSS is pretty straightforward.
Install Tailwind
Everything in this section can be found in Tailwind's documentation. If anything is unclear in this walkthrough or something isn't working be sure to check out their docs.
The first thing we'll need to do is open a new terminal tab or window and install Tailwind by running the following command in the root of our Shopify theme.
bash
Create Tailwind config file
Once Tailwind is installed you can run the following command to create the tailwind.config.js
file. This is the file that will contain all of your custom Tailwind configurations.
bash
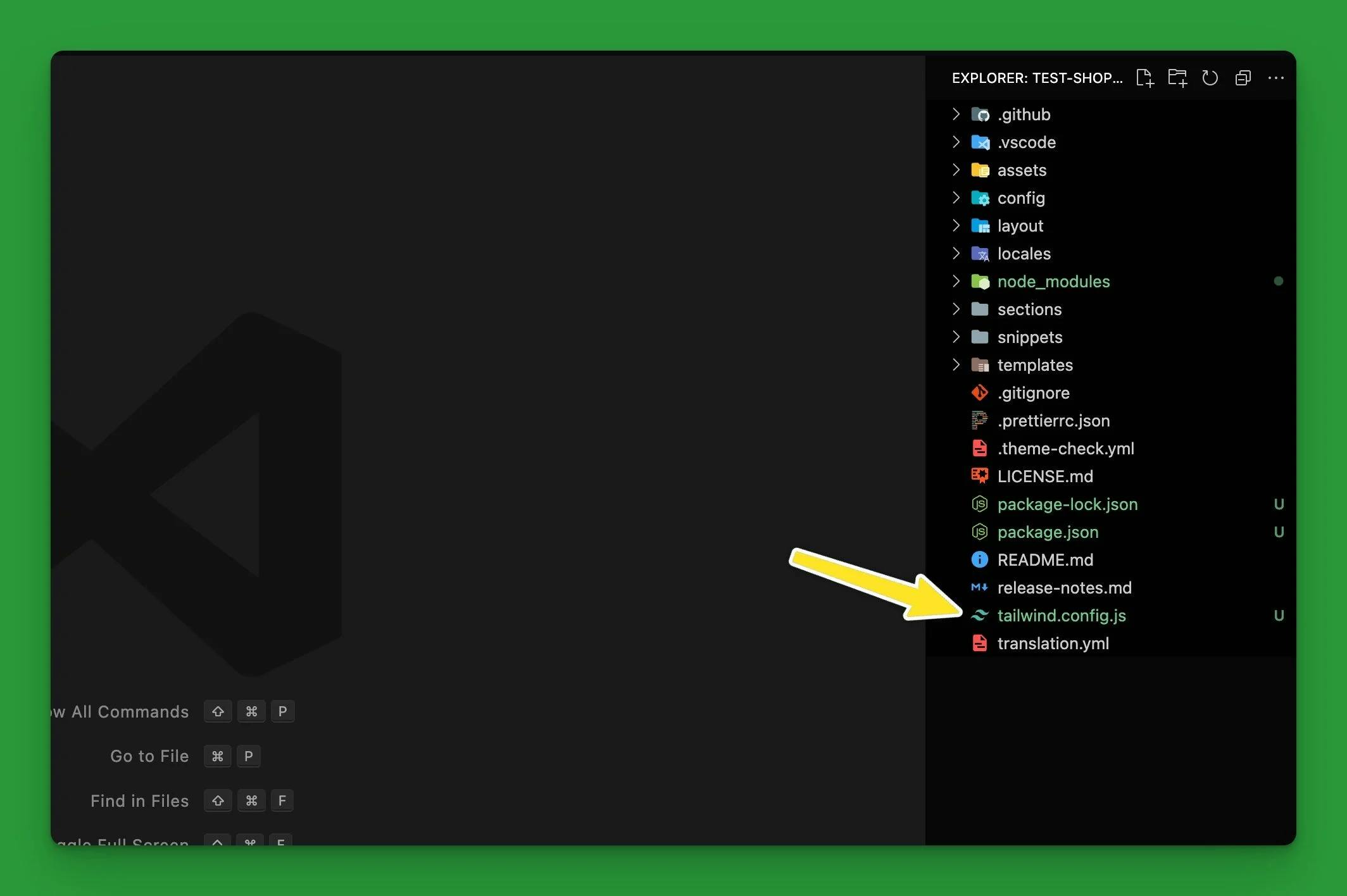
Next we'll update the tailwind.config.js
file and tell it which files to watch by updating the content
array to include all the .liquid
files in our theme.
tailwind.config.js
Create css files
Now we'll add a style.css
file in the root of our theme, next to the tailwind.config.js
file that we just added, and add the following @tailwind
directives.
style.css
Next we can run the follow npx
command to build our compiled css file. The --watch
flag will tell npx to continue to watch our files so we don't have to rerun the command every time we add or remove tailwind classes in our liquid templates.
bash
Make sure you replace style.css
and ./assets/style.css
if you've named your files something else.
Now we just need to make sure that our theme is loading our new ./assets/style.css
file. We can do this by adding a link to that file inside the <head>
in our theme.liquid
file.
theme.liquid
This will load the style.css
file from the ./assets
directory. To make sure your theme is loading the css file correctly you can add a tailwind class to an element and make sure it shows up on the frontend.
I usually like to add the class bg-red-500
to the header or body element.
Installing concurrently
Rather than having two separate terminal windows open, my preferred method for running the shopify dev server and npm tailwindcss command at the same time is to use concurrently
. This allows you to run multiple commands together in the same terminal window.
To get started add concurrently to your devDependencies.
bash
Next you can add the following to your package.json
scripts.
package.json
Now you can run npm run dev
, or yarn dev
if you're using yarn, to start your shopify dev server and run the npx tailwindcss command in the same terminal window.
npm:dev:*
will tell concurrently to run all the scripts that have the dev:
prefix.
Wrapping up
We've successfully added Tailwind CSS to our Shopify theme. Now we can get to work on building our next e-commerce empire. If you have any questions or you need help building that empire, feel free to shoot me a message!